There are different ways to control audio. MediaPlayer is good way. We see how to use MediaPlayer for control audio.
Download code
First step is to create file which name is raw in res/
Then in raw we must paste a song in mp3 format.
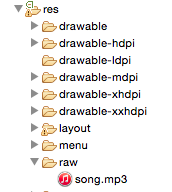
In order to use MediaPlayer , we have to call a static Method create() of this class. This method returns an instance of MediaPlayer class. Its syntax is as follows:
MediaPlayer mMediaPlayer = MediaPlayer.create(this, R.raw.song);
The second parameter is the name of the song that you want to play. You have to make a new folder under your project with name raw and place the music file into it.
Once you have created the Mediaplayer object you can call some methods to start or stop the music. These methods are listed below.
mMediaPlayer.start();
mMediaPlayer.pause();
you can read more about this methods in this link
Now We see simple example, First we need this strings
<string name="app_name">MediaPlayer</string>
<string name="now_play">Now Playing:</string>
<string name="inital_time">0 min, 0 sec</string>
<string name="pausing_sound">Pausing sound</string>
<string name="playing_sound">Playing sound</string>
<string name="action_song">Song</string>
In our activity_main.xml we need couple of textViews and imageButtons.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<ImageView
android:id="@+id/imageView_profile"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:src="@drawable/profile" />
<LinearLayout
android:id="@+id/linear_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:layout_marginBottom="14dp"
android:orientation="horizontal" >
<ImageButton
android:id="@+id/imageButton_media_rew"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="rewind"
android:src="@android:drawable/ic_media_rew" />
<ImageButton
android:id="@+id/imageButton_pause"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="14dp"
android:src="@android:drawable/ic_media_pause" />
<ImageButton
android:id="@+id/imageButton_play"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="14dp"
android:src="@android:drawable/ic_media_play" />
<ImageButton
android:id="@+id/imageButton_media_ff"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="14dp"
android:onClick="forward"
android:src="@android:drawable/ic_media_ff" />
</LinearLayout>
<SeekBar
android:id="@+id/seekBar1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_above="@+id/linear_layout"
android:layout_toLeftOf="@+id/textView2"
android:layout_toRightOf="@+id/textView1" />
<TextView
android:id="@+id/textView_start_min"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignTop="@+id/seekBar1"
android:text="@string/inital_time"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/textView_finish_min"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignTop="@+id/seekBar1"
android:text="@string/inital_time"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/textView_title_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/now_play"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/textView_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/textView_title_name"
android:layout_alignBottom="@+id/textView_title_name"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@+id/textView_title_name" />
</RelativeLayout>
The last step in MainActivity
package com.thedeveloperworldisyours.mediaplayer;
import java.util.concurrent.TimeUnit;
import android.app.Activity;
import android.app.ProgressDialog;
import android.content.Intent;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.os.Handler;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.ImageButton;
import android.widget.SeekBar;
import android.widget.TextView;
import android.widget.Toast;
import com.thedeveloperworldisyours.mediaplayer.utils.Constants;
public class MainActivity extends Activity implements OnClickListener {
public TextView mSongName, mStartTimeField, mEndTimeField;
private MediaPlayer mMediaPlayer;
private double mStartTime = 0;
private double mFinalTime = 0;
private Handler mMyHandler = new Handler();;
private int mForwardTime = 5000;
private int mBackwardTime = 5000;
private SeekBar mSeekbar;
private ImageButton mPlayButton, mPauseButton;
public static int mOneTimeOnly = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mSongName = (TextView) findViewById(R.id.textView_name);
mStartTimeField = (TextView) findViewById(R.id.textView_start_min);
mEndTimeField = (TextView) findViewById(R.id.textView_finish_min);
mSeekbar = (SeekBar) findViewById(R.id.seekBar1);
mPlayButton = (ImageButton) findViewById(R.id.imageButton_play);
mPauseButton = (ImageButton) findViewById(R.id.imageButton_pause);
mSongName.setText(Constants.NAME_SONG);
mMediaPlayer = MediaPlayer.create(this, R.raw.song);
mSeekbar.setClickable(false);
mPauseButton.setEnabled(false);
mPlayButton.setOnClickListener(this);
mPauseButton.setOnClickListener(this);
}
public void play(View view) {
Toast.makeText(getApplicationContext(),
getString(R.string.playing_sound), Toast.LENGTH_SHORT).show();
mMediaPlayer.start();
mFinalTime = mMediaPlayer.getDuration();
mStartTime = mMediaPlayer.getCurrentPosition();
if (mOneTimeOnly == 0) {
mSeekbar.setMax((int) mFinalTime);
mOneTimeOnly = 1;
}
mEndTimeField.setText(String.format(
"%d min, %d sec",
TimeUnit.MILLISECONDS.toMinutes((long) mFinalTime),
TimeUnit.MILLISECONDS.toSeconds((long) mFinalTime)
- TimeUnit.MINUTES.toSeconds(TimeUnit.MILLISECONDS
.toMinutes((long) mFinalTime))));
mStartTimeField.setText(String.format(
"%d min, %d sec",
TimeUnit.MILLISECONDS.toMinutes((long) mStartTime),
TimeUnit.MILLISECONDS.toSeconds((long) mStartTime)
- TimeUnit.MINUTES.toSeconds(TimeUnit.MILLISECONDS
.toMinutes((long) mStartTime))));
mSeekbar.setProgress((int) mStartTime);
mMyHandler.postDelayed(UpdateSongTime, 100);
mPauseButton.setEnabled(true);
mPlayButton.setEnabled(false);
}
private Runnable UpdateSongTime = new Runnable() {
public void run() {
mStartTime = mMediaPlayer.getCurrentPosition();
mStartTimeField.setText(String.format(
"%d min, %d sec",
TimeUnit.MILLISECONDS.toMinutes((long) mStartTime),
TimeUnit.MILLISECONDS.toSeconds((long) mStartTime)
- TimeUnit.MINUTES.toSeconds(TimeUnit.MILLISECONDS
.toMinutes((long) mStartTime))));
mSeekbar.setProgress((int) mStartTime);
mMyHandler.postDelayed(this, 100);
}
};
public void pause(View view) {
Toast.makeText(getApplicationContext(),
getString(R.string.pausing_sound), Toast.LENGTH_SHORT).show();
mMediaPlayer.pause();
mPauseButton.setEnabled(false);
mPlayButton.setEnabled(true);
}
public void forward(View view) {
int temp = (int) mStartTime;
if ((temp + mForwardTime) <= mFinalTime) {
mStartTime = mStartTime + mForwardTime;
mMediaPlayer.seekTo((int) mStartTime);
} else {
Toast.makeText(getApplicationContext(),
"Cannot jump forward 5 seconds", Toast.LENGTH_SHORT).show();
}
}
public void rewind(View view) {
int temp = (int) mStartTime;
if ((temp - mBackwardTime) > 0) {
mStartTime = mStartTime - mBackwardTime;
mMediaPlayer.seekTo((int) mStartTime);
} else {
Toast.makeText(getApplicationContext(),
"Cannot jump backward 5 seconds", Toast.LENGTH_SHORT)
.show();
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.imageButton_play:
play(v);
break;
case R.id.imageButton_pause:
pause(v);
break;
default:
break;
}
}
}
Download code