How to use GraphQL with Retrofit on Android?
Have you use GraphQL on Android?
GraphQL is a query language for APIs and a runtime for fulfilling those queries with your existing data. GraphQL provides a complete and understandable description of the data in your API, gives clients the power to ask for exactly what they need and nothing more, makes it easier to evolve APIs over time, and enables powerful developer tools.
Apollo Android is a GraphQL client that generates Java and Kotlin models from GraphQL queries. These models give you a type-safe API to work with GraphQL servers. Apollo helps you keep your GraphQL query statements together, organized, and easy to access.
You can choose to use Apollo in android when you query is static. But what happen when the query is dynamic query?
We recommend you to use GraphQL with retrofit.
In your manifest to add
<uses-permission android:name="android.permission.INTERNET"/>
Your dependencies
// Kotlin Coroutines
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.3.4'
//OkHttp
implementation ("com.squareup.okhttp3:okhttp:3.12.12"){
force = true //API 19 support
}
implementation 'com.squareup.okhttp3:logging-interceptor:3.12.12'
//retrofit
implementation "com.squareup.retrofit2:retrofit:2.7.1"
implementation "com.squareup.retrofit2:converter-scalars:$2.7.1"
Also Java 8 compatibility
android {
...
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = "1.8"
}
}
With the service
import retrofit2.Response
import retrofit2.http.Body
import retrofit2.http.Headers
import retrofit2.http.POST
interface GraphQLService {
@Headers("Content-Type: application/json")
@POST("/")
suspend fun postDynamicQuery(@Body body: String): Response
}
you can create a object
import retrofit2.Retrofit
import retrofit2.converter.scalars.ScalarsConverterFactory
object GraphQLInstance {
private const val BASE_URL: String = "http://192.155.1.55:2000/"
val graphQLService: GraphQLService by lazy {
Retrofit
.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(ScalarsConverterFactory.create())
.build().create(GraphQLService::class.java)
}
}
In the activity you can create this method
private fun post(userId: String){
val retrofit = GraphQLInstance.graphQLService
val paramObject = JSONObject()
paramObject.put("query", "query {users(userid:$userId){username}}")
GlobalScope.launch {
try {
val response = retrofit.postDynamicQuery(paramObject.toString())
Log.e("response", response.body().toString())
}catch (e: java.lang.Exception){
e.printStackTrace()
}
}
}
You can check GraphQL with Retrofit on Android in the example in GitHub
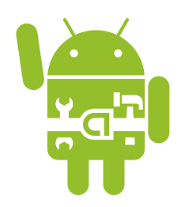