Edit
We can edit a PDF, we have to create a new copy from original.
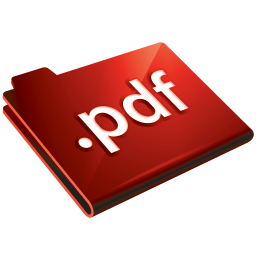
private static String sInPutfile = "Android/data/Example.pdf";
private static String sOutPutFile = "Android/data/ExampleCopy.pdf";
Then we have to PdfReader and PdfStamper, you can check the library iText.
private void editPDF(String string) {
try {
FileInputStream is = new FileInputStream(mPdfFile);
PdfReader pdfReader = new PdfReader(is);
PdfStamper pdfStamper = new PdfStamper(pdfReader,
new FileOutputStream(mPdfFileOutPut));
for (int i = 1; i <= pdfReader.getNumberOfPages(); i++) {
//create content from pdfStamper
PdfContentByte content = pdfStamper.getUnderContent(i);
// Text over the existing page
BaseFont bf = BaseFont.createFont(BaseFont.HELVETICA,
BaseFont.WINANSI, BaseFont.EMBEDDED);
content.beginText();
//set the font
content.setFontAndSize(bf, 18);
content.showTextAligned(PdfContentByte.ALIGN_LEFT, string, 430, 15, 0);
content.endText();
}
pdfStamper.close();
} catch (IOException e) {
e.printStackTrace();
} catch (DocumentException e) {
e.printStackTrace();
}
}
And we can edit the PDF generated other PDF in android.
Example in Github